Web Scraping made easy: The Importance of Data Parsing
Suciu Dan on Apr 26 2023
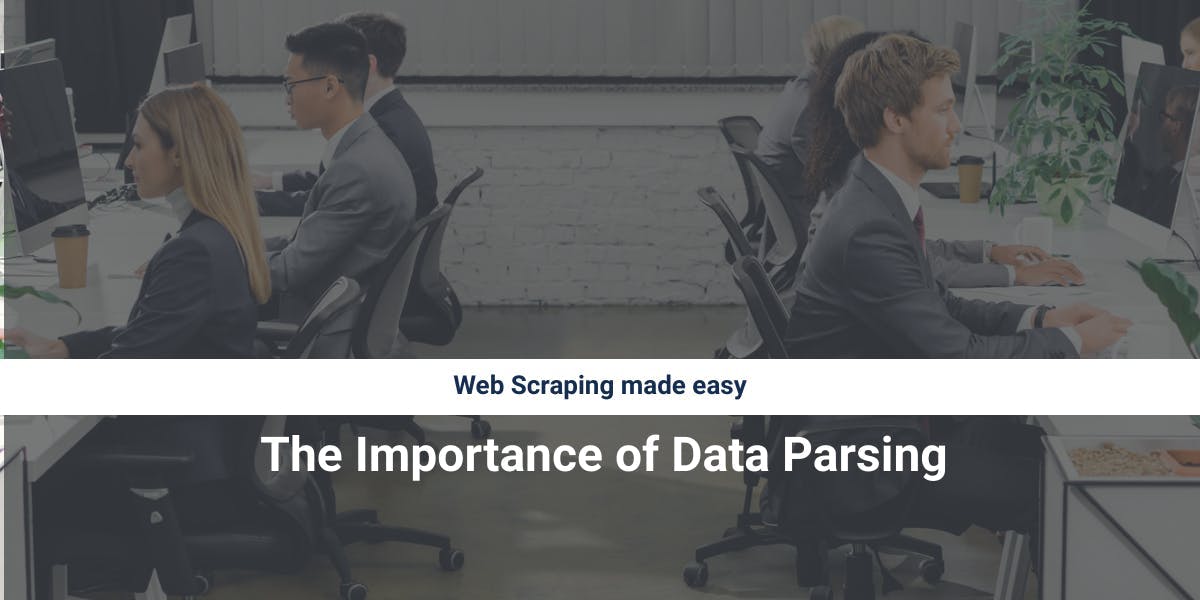
Data parsing is the process of analyzing and transforming structured or unstructured data into a more specific format that various software systems can use and understand.
To make this concept easier to understand, here are some examples of parsers:
- CSV parsers: for parsing and converting CSV (Comma Separated Values) files into more usable formats
- JSON parsers: for parsing and converting JSON (JavaScript Object Notation) data into more usable formats
- Regex parsers: for parsing and extracting specific patterns of text using regular expressions
- Compilers: for parsing and converting code written in one programming language into machine-readable code in another programming language
- SQL Parsers: for analyzing and interpreting a SQL query, carrying out the command, and returning the results
When it comes to web scraping, data parsing is essential. Websites are made up of HTML, a great markup language for displaying information on a screen but not so great for machines to read.
When we scrape a website, we're grabbing a big string of HTML. To do anything useful with that information, we need to parse it.
This will cover the importance of data parsing in web scraping, as well as the different types of data parsers available, such as HTML parsing libraries for various programming languages, regular expressions, and building your parser.
Creating a Data Parser
A good data parser can extract relevant information from an HTML document based on pre-defined rules, regardless of the type of parser used. The parsing process consists of two main steps: lexical analysis and syntactic analysis.
Lexical analysis is the process of analyzing individual words and symbols in a document and breaking them down into smaller, more manageable pieces.
This involves tokenization, which is the process of breaking a document down into individual tokens, such as keywords, symbols, and numbers.
Let’s take a look at this simple HTML document:
<html>
<head>
<title>Scraping</title>
</head>
<body>
<h1>Welcome to my scraping page</h1>
<p>This is a paragraph.</p>
<ul>
<li>First Scraping Item</li>
<li>Second Scraping Item</li>
</ul>
</body>
</html>
The lexical analysis process would tokenize this document into individual elements such as:
- `<html>`
- `<head>`
- `<title>`
- `Scraping`
- `</title>`
- `<body>`
- `<h1>`
- `Welcome to my scraping page`
- `</h1>`
- [...]
- `</body>`
- `</html>`
This way, each element of the HTML document gets split down into smaller, more manageable tokens that can be further analyzed and processed.
Syntactic analysis is the process of analyzing the structure of a document and determining how the individual tokens relate to each other. This involves identifying patterns and structures in the data and using this information to create a tree-like structure called a parse tree.
For example, the <html> tag is the root element, and it contains the <head> and <body> elements. Within the <head> element, there is a <title> element, and within the <body> element, there are <h1>, <p>, and <a> elements.
By identifying these elements and their relationships, you can construct a parse tree, with the <html> element as the root, <head> and <body> as its children, and so on.
You can use the parse tree to extract specific data from the HTML document, such as the text within the <title> element, or the href attribute of the <a> element.
Best HTML Parsing Libraries
In this section, we will explore some of the most popular HTML parsing libraries available for different programming languages. These libraries make it easy to extract structured data from an HTML document and can be a great starting point for your web scraping project.
From Python's Scrapy and BeautifulSoup, to NodeJS's Cheerio and Java's JSoup, we will take a look at each library and provide examples of how to use them.
Whether you are a beginner or an experienced developer, this section will give you a solid understanding of the options available to you when working with HTML data.
Let’s start!
Cheerio
Cheerio is a JavaScript library that allows developers to parse, manipulate, and navigate the DOM of an HTML or XML document, like how jQuery works. This article goes into much more detail about Cheerio and talks about different use cases.
Here’s a simple cheerio implementation:
const cheerio = require('cheerio');
const $ = cheerio.load('<h2 class="title">Hello, World!</h2>');
console.log($('h2').text())
Running this code will return the following output:
Hello, World!
Scrapy and BeautifulSoup
Scrapy and BeautifulSoup are libraries for web scraping in Python.
Scrapy is a powerful web scraping framework that allows you to extract structured data from websites by using selectors or XPath expressions.
Here’s a basic Scrapy example:
import scrapy
class QuotesSpider(scrapy.Spider):
name = "quotes"
def start_requests(self):
urls = [
'https://quotes.toscrape.com/page/1/',
'https://quotes.toscrape.com/page/2/',
]
for url in urls:
yield scrapy.Request(url=url, callback=self.parse)
def parse(self, response):
page = response.url.split("/")[-2]
filename = f'quotes-{page}.html'
with open(filename, 'wb') as f:
f.write(response.body)
self.log(f'Saved file {filename}')
You can run the code with this command:
scrapy crawl quotes
BeautifulSoup is a library that allows you to parse HTML and XML documents and extract data from them in a way like how a web browser does.
Here’s a simple BeautifulSoup implementation:
from bs4 import BeautifulSoup
html_doc = """<html><head><title>Scraper</title></head>
<body>
<h1 class="title">Hello, World!</h1>
</body>"""
soup = BeautifulSoup(html_doc, 'html.parser')
print(soup.title.string)
Both of these libraries provide a simple and convenient API for traversing, searching, and modifying the content of web pages, and have a perfect use in web scraping projects.
JSoup
If your programming language of choice is Java, JSoup is a data parser that provides a convenient API for extracting and manipulating data, using the best of DOM, CSS, and jQuery-like methods.
This allows you to parse and navigate HTML documents, and change the contents of a document using a simple, intuitive API. The library is a perfect fit for web scraping, web crawling, and data extraction projects.
Here’s a simple implementation of JSoup for extracting the text from the title tag:
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
public class JSoupExample {
public static void main(String[] args) {
String html = "<html><head><title>Example Title</title></head>"
+ "<body>Hello, World!</body></html>";
Document doc = Jsoup.parse(html);
// Get the title of the document
String title = doc.title();
System.out.println("Title: " + title);
}
}
Nokogiri
Nokogiri is a library for Ruby that provides an easy-to-use interface for parsing and searching XML and HTML documents and supports XPath and CSS selectors, making it used for web scraping and data extraction tasks.
For a more comprehensive analysis of the data parser libraries in the Ruby ecosystem, you can read this article.
Use this command to install the nokogiri gem:
gem install nokogiri
The next code sample is a simple nokogiri implementation:
require "nokogiri"
html = "<!DOCTYPE html><html><head><title>Hello, World!</title></head><body>Hello, World!</body></html>"
parsed_data = Nokogiri::HTML.parse(html)
puts parsed_data.title
Regular Expressions
Regular expressions, also known as regex, are a powerful tool for matching patterns in strings. They are often used for text-processing tasks such as searching, validating, and extracting information from a document.
You can use regular expressions to extract information from HTML by searching for specific patterns, like extracting email addresses or headings from an HTML document.
For example, to extract all the URLs from an HTML document, you can use the following regular expression:
/https?:\/\/[\w\.-]+\.[a-z]+/gi
This expression will match any string that starts with "http" or "https" followed by a ":" and two slashes, then any combination of word characters, dots, and hyphens, followed by a dot and one or more lowercase letters. The "gi" flag makes the search global and case-insensitive.
Using regular expressions can be a bit tricky, as the syntax can be complex, and getting the pattern exactly right can take some trial and error. There are also some sites like Regex101 or Rubular, that can help you test and debug your regular expressions.
If you don't want to use the available libraries or regular expressions, you can always build your parser.
Building your parser can be a great way to gain a deeper understanding of the data you're working with and can also be a good option if you have specific requirements that aren't met by existing libraries or tools.
Building a parser
Building your parser can be a challenging task, but it can also be a rewarding one. The process of building a parser involves creating a set of rules and instructions that specify how the data gets parsed and organized.
You can do this by using a variety of techniques, such as regular expressions, state machines, and recursive descent parsing.
When building a parser, it's crucial to have a thorough understanding of the data's structure and format to be able to design an appropriate set of rules and instructions for the parser. Choosing an appropriate programming language is also an important consideration.
One of the advantages of building your parser is that you can tailor it to the data and use case at hand. This can result in a more efficient and effective parser, compared to using a generic library or tool.
Additionally, building your parser can also be a great learning experience, as it allows you to gain a deeper understanding of the underlying concepts and techniques of data parsing.
Building a parser from scratch comes with disadvantages as well:
- It can be time-consuming and need a significant amount of effort to design and implement, especially if you're not familiar with parsing algorithms and data structures.
- It can be difficult to get your parser to perform as well as existing libraries or tools that have been optimized for performance.
- It can be hard to maintain and update the parser over time if the data format or structure changes.
- It might be hard to debug or find errors in your code, especially if you are not familiar with the parsing process.
- It could be prone to errors and bugs, which could lead to the parser not working as expected.
- In the case of complex parsing, it could be hard to implement all the rules and edge cases.
- it might not be as efficient as pre-existing libraries and tools, as they have been optimized and used by many people.
In summary, building a custom parser from scratch has its own set of disadvantages, such as high development time, high maintenance cost, and high risk of errors. It's generally recommended to use existing libraries or tools or to use regular expressions if they can meet the specific requirements of your use case.
Schema.org metadata
Parsing schema.org metadata is a way to extract structured data from web pages using web schema standards. The community behind schema.org manages these standards and promotes the use of schema for structured data on the web.
Parsing schema metadata can be useful for various reasons, such as finding updated information on events, or for researchers gathering data for studies. Additionally, websites that aggregate data like real-estate listings, job postings, and weather forecasts can also benefit from parsing schema data.
There are different formats of schema you can use, including JSON-LD, RDFa, and Microdata.
JSON-LD (JavaScript Object Notation for Linked Data) is a format for encoding linked data using JSON. The design of this standard makes it easy for humans to read and write and for machines to parse and generate.
Here’s how JSON-LD would look for a web page about a book:
<script type="application/ld+json">
{
"@context": "http://schema.org",
"@type": "Book",
"name": "The Adventures of Tom Sawyer",
"author": "Mark Twain",
"datePublished": "1876-12-01",
"description": "The Adventures of Tom Sawyer is a novel about a young boy growing up along the Mississippi River in the mid-1800s. It is a classic of American literature and has been loved by generations of readers.",
"publisher": "Penguin Books",
"image": "https://www.example.com/images/tom_sawyer.jpg"
}
</script>
World Wide Web Consortium (W3C) recommendation is RDFa, or Resource Description Framework in Attributes, used to embed RDF statements in XML and HTML.
You can find below how the RDFa would look inside an HTML page. You can notice how tag attributes are used to store the extra data.
<!DOCTYPE html>
<html>
<head>
<title>RDFa Example</title>
</head>
<body>
<div about="http://example.com/books/the-great-gatsby" typeof="schema:Book">
<h1 property="schema:name">The Great Gatsby</h1>
<div property="schema:author" typeof="schema:Person">
<span property="schema:name">F. Scott Fitzgerald</span>
</div>
<div property="schema:review" typeof="schema:Review">
<span property="schema:author" typeof="schema:Person">
<span property="schema:name">John Doe</span>
</span>
<span property="schema:reviewBody">
A classic novel that explores themes of wealth, love, and the decline of the American Dream.
</span>
<span property="schema:ratingValue">4.5</span>
</div>
</div>
</body>
</html>
Microdata is a WHATWG HTML specification that is used to nest metadata inside existing content on web pages and can use schema.org or custom vocabularies.
Here is an example of Microdata in HTML:
<div itemscope itemtype="http://schema.org/Product">
<span itemprop="name">Shiny new gadget</span>
<img itemprop="image" src="shinygadget.jpg" alt="A shiny new gadget" />
<div itemprop="offerDetails" itemscope itemtype="http://schema.org/Offer">
<span itemprop="price">$19.99</span>
<link itemprop="availability" href="http://schema.org/InStock" />
</div>
</div>
There are many tools available to parse schema across different languages, such as Extruct from Zyte and RDFLib library, making it easy to extract structured data from web pages using web schema standards.
Premium parsers
So far, we discussed the fundamentals of data parsing, including the underlying concepts of lexical and syntactic analysis. We also examined various open-source libraries for data parsing, the use of regular expressions, building a parser from the ground up, and parsing data using schema.org.
You can always rely on a web parser like WebScrapingAPI SERP API or Amazon API. These web parsers allow you to scrape data in real time without having to worry about maintenance, code, or infrastructure.
There are several advantages to using a premium web parser, including:
- Reliability: Web parsers are generally more stable and reliable than free or open-source alternatives, which can be prone to bugs and errors.
- Speed: Web parsers are optimized for speed and performance, allowing you to extract data fast and efficiently.
- Scalability: Web parsers can handle large volumes of data and high levels of traffic, making them suitable for large-scale scraping and data extraction projects.
- Advanced features: Web parsers often include advanced features and functionalities, such as IP rotation, user agent spoofing, and CAPTCHA solving, that can help you to bypass anti-scraping measures and access blocked websites.
- Support and maintenance: Web parsers come with customer support and regular software updates, ensuring that you have access to the latest features and bug fixes.
But let’s be honest: premium web parsers are not bringing only advantages. Here are some disadvantages:
- Cost: Premium web parsers may have a higher cost associated with them compared to open-source options
- Limited customization: The functionality of a premium web parser may be more limited compared to building your parser
- Dependence on the service: If the service goes down or experiences any issues, it can disrupt your ability to parse data
- Limited control over data: With a premium web parser, you may have less control over the data you can access and process
- Dependence on the provider's data sources: The quality and relevance of the data provided by the premium web parser may be limited by the provider's data sources.
Conclusion
This article has provided a comprehensive overview of data parsing, including the parsing process, different types of HTML parsing libraries, and how to use schema.org metadata for improved SEO.
We also highlighted the advantages and disadvantages of building a custom parser, using regular expressions, and using existing tools.
A key takeaway is that data parsing is a crucial step in web scraping and data analysis as it allows you to extract and organize information in a useful way.
To help you get started, you can try our SERP API, a premium web scraping tool that can help you easily extract data from search engines. If you're interested in trying it out, don't hesitate to sign up for our 14-day free trial.
News and updates
Stay up-to-date with the latest web scraping guides and news by subscribing to our newsletter.
We care about the protection of your data. Read our Privacy Policy.
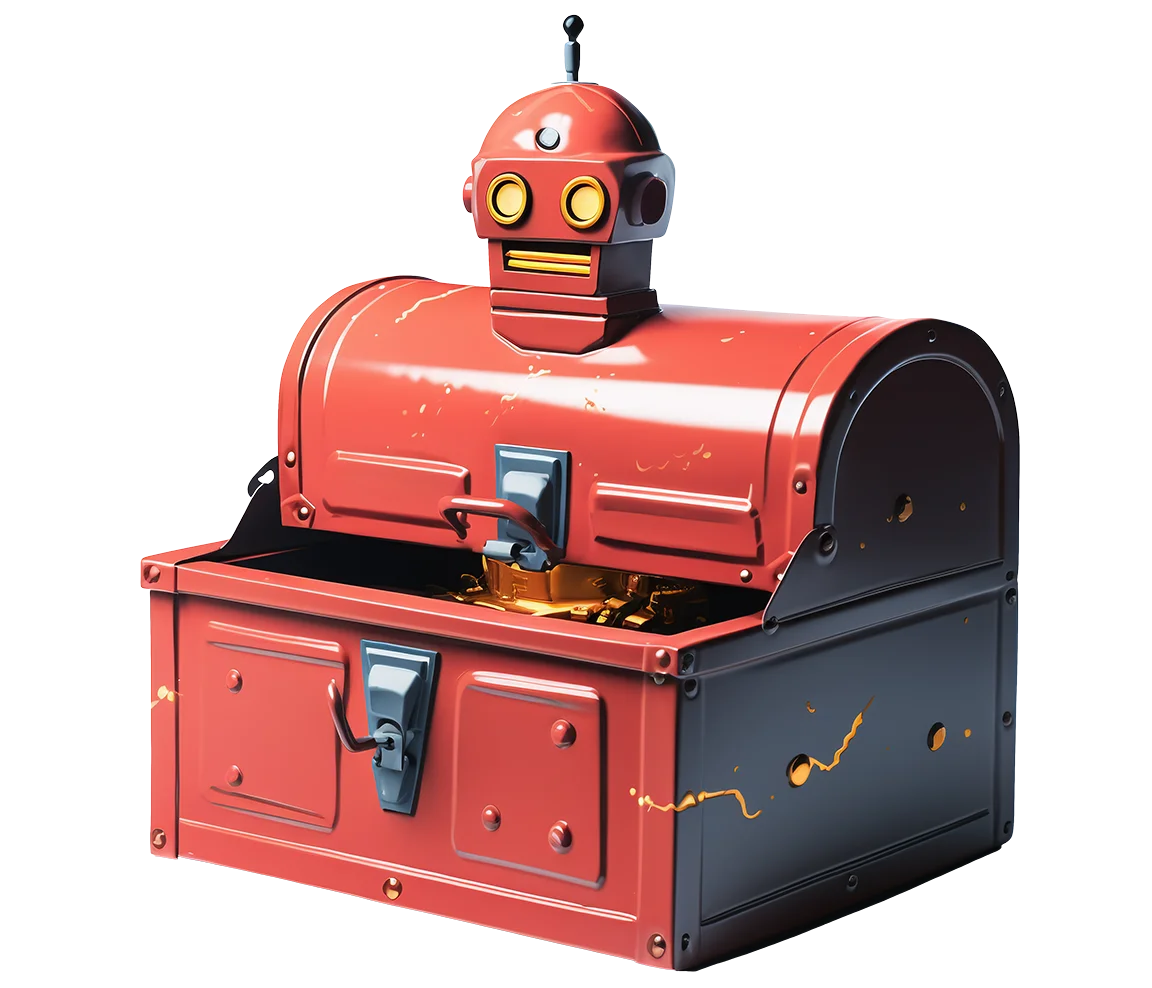
Related articles

Scrape Amazon efficiently with Web Scraping API's cost-effective solution. Access real-time data, from products to seller profiles. Sign up now!

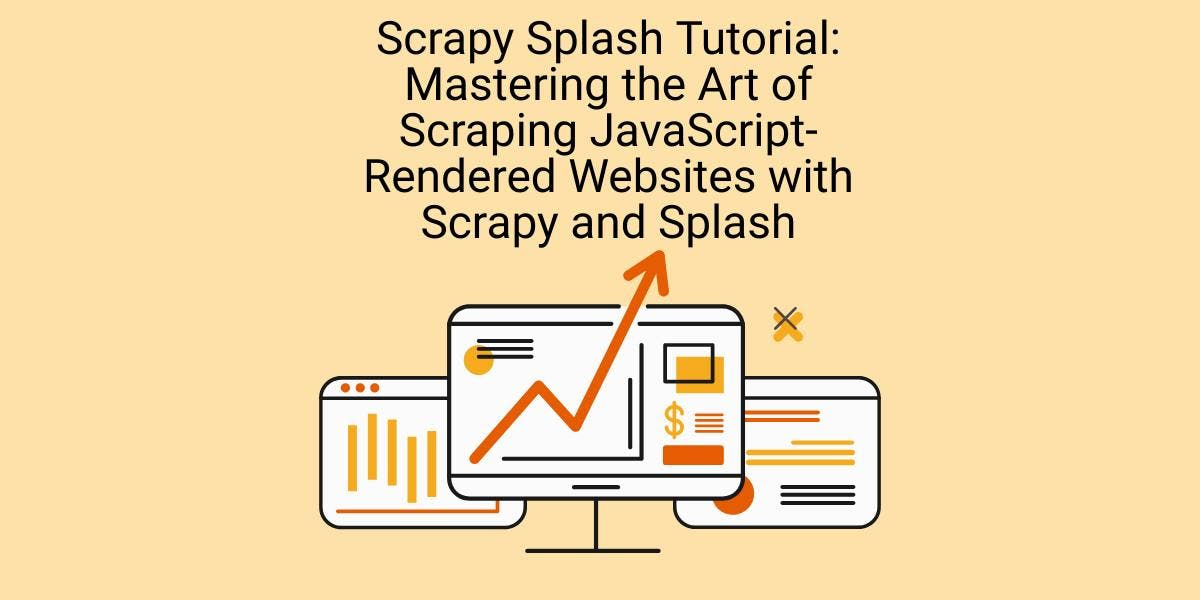
Learn how to scrape dynamic JavaScript-rendered websites using Scrapy and Splash. From installation to writing a spider, handling pagination, and managing Splash responses, this comprehensive guide offers step-by-step instructions for beginners and experts alike.

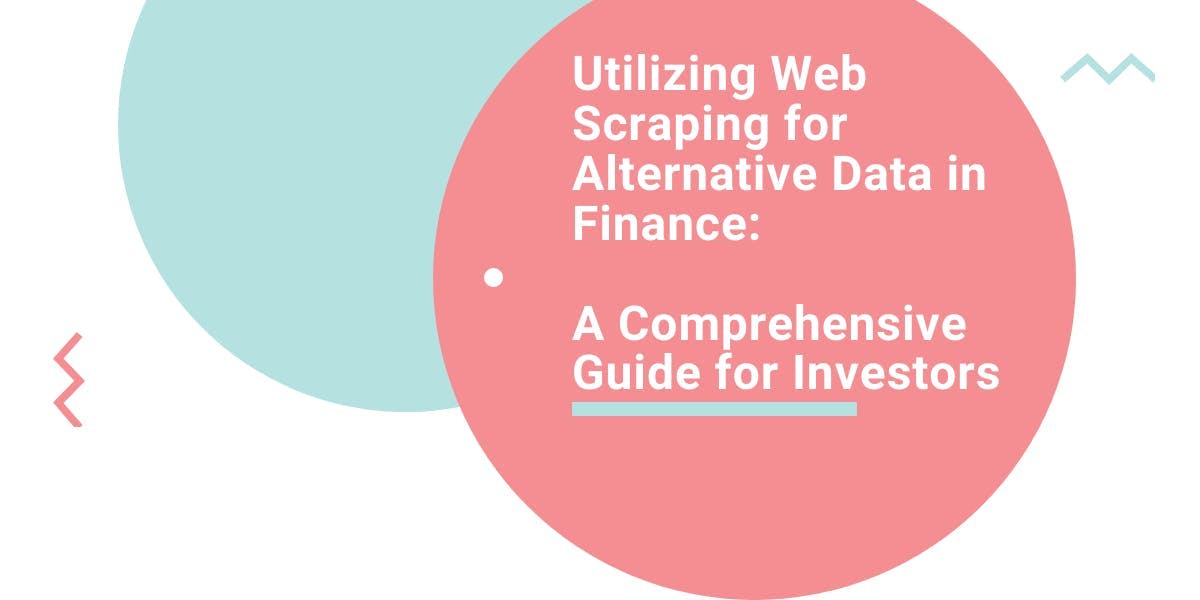
Explore the transformative power of web scraping in the finance sector. From product data to sentiment analysis, this guide offers insights into the various types of web data available for investment decisions.
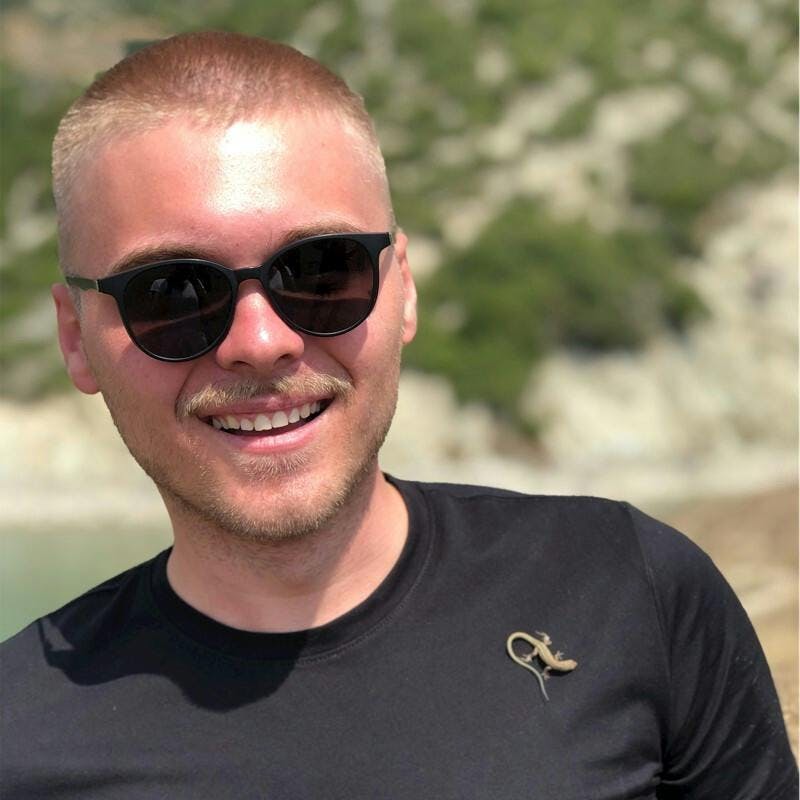