The Ultimate Guide to Ruby Libraries for Parsing HTML & XML
Suciu Dan on Feb 02 2023
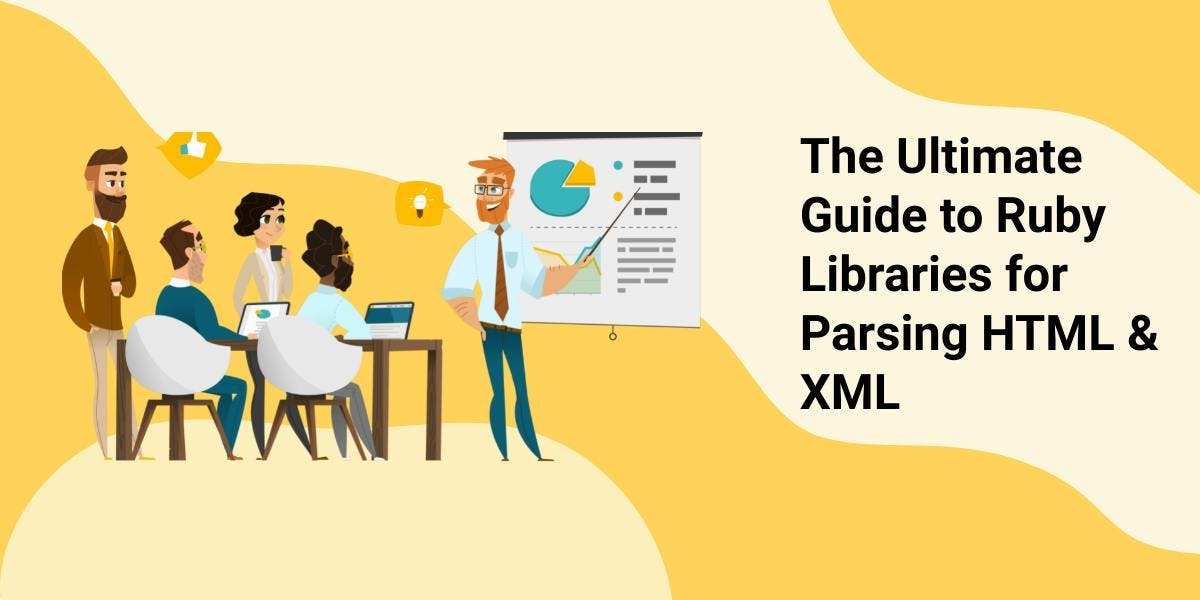
Web scraping, or extracting data from the web, involves reading and processing content from HTML and XML documents. To make this task easier, developers use specialized libraries called parsers.
The Ruby community offers a wide range of options when it comes to Ruby HTML parsers, and choosing the right one for your project can be a daunting task. To help you make an informed decision, here are a few key factors to consider when selecting a parser:
- Being open-source and freely available for use.
- The level of support for different HTML and XML standards.
- Having comprehensive documentation and tutorials to help developers easily get started.
- The ability to handle different types of encodings, especially when dealing with non-latin languages.
- Having a lightweight and easy-to-use API, making it easy to navigate and search through HTML and XML documents.
- The level of error handling and validation provided by the library.
- Having a strong and active community that provides support and resources.
- The size and memory footprint of the library.
- Having good performance, especially when working with large files.
- The level of support for XML namespaces, if dealing with documents that use them.
- Being actively maintained to ensure compatibility with the latest versions of Ruby and to receive bug fixes.
- The level of extendability or customization options that the library offers.
This article will take a closer look at six popular Ruby libraries for parsing HTML and XML, and evaluate them based on the aforementioned criteria to help you find the perfect tool for your web scraping needs.
Nokogiri
Nokogiri is a popular and powerful library for parsing and searching XML and HTML documents in Ruby. It has a clean and simple API and it's built on top of libxml2, a well-established C library for parsing XML.
Gem Command
gem install nokogiri
Code Samples
require "nokogiri"
html = "<!DOCTYPE html><html><head><title>Hello, World!</title></head><body>Hello, World!</body></html>"
parsed_data = Nokogiri::HTML.parse(html)
puts parsed_data.title
Pros and Cons
Here are some of the pros and cons of using Nokogiri:
Pros
- It’s widely considered to be the most popular and most used parser for Ruby
- It’s very fast and efficient, thanks to its use of libxml2 as a parsing engine. It can handle large documents with ease.
- It has a simple and user-friendly API that makes it easy to navigate and search through XML and HTML documents.
- It supports both XML and HTML documents, which allows you to use the same library for parsing different types of documents.
- It has a rich set of methods for searching and manipulating elements in a document, which makes it easy to extract the information you need. You can extract data using CSS selectors or XPath.
- It can parse malformed HTML documents
- It is compatible with different Ruby versions and it's actively maintained.
- It also supports both SAX (Simple API for XML) and DOM (Document Object Model) parsers
Cons
- Some of the parsing tasks might require a deep understanding of the DOM structure, which can be difficult to learn if the developer is not familiar with it.
- It might require more memory compared to other libraries like Ox.
- It may face difficulties parsing documents protected by authentication, for example a website that requires a username and password to access.
- It is not thread-safe, so you need to take extra care if you're planning to use it in a multithreaded environment.
- It is not well-suited for passing documents with dynamically loaded content via JavaScript, such as with AJAX.
Ox
Ox, or Optimized XML, is a powerful and efficient library for parsing and manipulating XML and JSON documents in Ruby.
The library is implemented in C for better performance and memory efficiency. Ox uses a pull-parser approach to parse the document, which allows it to parse large files with less memory usage than a DOM based parser.
Some of the ways that Ox processes XML documents are:
- As a generic XML parser and writer: Ox can read and write XML documents, providing methods for searching and manipulating elements in the document.
- As a fast Object/XML Marshaller: Ox can convert XML documents to Ruby objects and vice versa. This feature allows for easy data serialization and deserialization.
- As a stream SAX parser: Ox can parse XML in a streaming manner which is suitable for large files and provides a fast way to handle the XML events.
Gem Command
gem install ox
Code Samples
require "ox"
doc = Ox.parse(%{
<?xml version="1.0"?>
<Payment>
<Shop>ikea</Shop>
<Amount>199.99</Amount>
<Date>2023-01-12</Date>
</Payment>
})
puts doc.Payment.Shop.text
Pros & Cons
Here are some pros and cons of using Ox:
Pros
- Ox is very fast and memory-efficient, thanks to its use of a pull-parser approach and the fact that it's implemented in C. This makes it well-suited for parsing large XML and JSON documents or working with streaming data
- Ox has a clean and simple API that makes it easy to use and understand
- Ox supports both JSON and XML, which allows you to use the same library for parsing different types of documents
- It has a built-in support for XML namespaces, which makes it easier to handle XML documents with namespaces.
- It is actively maintained and updated
Cons
- The API for searching and manipulating elements might be less rich compared to other libraries like Nokogiri or REXML
- Its community and support may not be as strong as those of more established libraries like Nokogiri
Oga
Oga is a modern and lightweight library for parsing and searching XML and HTML documents in Ruby. It uses a more modern approach compared to other libraries by using a pure-Ruby implementation, which means it doesn't have any dependencies on C libraries.
The library is suitable for small-medium size documents and doesn't require advanced features like XSLT or XML Schema validation.
Even though the library does not require any system libraries like libxml, to achieve better performance, Oga uses a small, native extension (C for MRI/Rubinius, Java for JRuby).
Gem Command
gem install oga
Code Samples
require "oga"
doc = Oga.parse_xml(%{
<?xml version="1.0"?>
<Payment>
<Shop>ikea</Shop>
<Amount>199.99</Amount>
<Date>2023-01-12</Date>
</Payment>
})
puts doc.at_xpath("Payment/Shop/text()")
Pros & Cons
Here are some pros and cons of using Oga:
Pros
- Oga has a simple and clean API, making it easy to navigate and search through XML and HTML documents.
- Pure-Ruby implementation makes it easy to install and run on different platforms and environments.
- Oga's API allows for parsing and querying documents in a multi-threaded environment safely, without concern for performance issues
- Oga is lightweight and easy to integrate with other libraries and modules.
- Oga has a low memory footprint.
Cons
- Oga lacks support for advanced features such as XPath, XSLT, or validation of XML documents against a DTD or XML Schema.
- Oga's features are limited compared to other libraries like Nokogiri, which may not be suitable for complex XML or HTML parsing tasks.
- Even though it’s maintained, it receives less updates compared to Nokogiri
LibXML Ruby
LibXML Ruby is a binding to the libxml2 C library, which is a well-established library for parsing and manipulating XML documents. The binding provides an interface to the functionality of libxml2, and it is used by several other popular libraries, including Nokogiri.
The library comes with advanced features like XPath support, DTD parsing, XSL Transformations, and more.
Gem Command
gem install libxml-ruby
Code Samples
require "xml"
doc = XML::Parser.string(%{
<?xml version="1.0"?>
<Payment>
<Shop>ikea</Shop>
<Amount>199.99</Amount>
<Date>2023-01-12</Date>
</Payment>
})
puts doc.parse.find('//Shop').first.content
Pros & Cons
Here is a list of its pros and cons:
Pros
- Provides a fast and efficient way to parse and manipulate XML and HTML documents in Ruby, thanks to its underlying C library.
- It supports multiple encoding types and can handle documents with complex structures and namespaces
- Provides support for XPath, which is a language that allows you to navigate and select elements from an XML document based on their properties and relationships.
- Supports XSLT transformations and DTD/XML schema validation
- Has a wide range of features and options that make it suitable for advanced use cases.
- It's well supported by the community, and it's a stable and well-documented library.
Cons
- It can consume more memory than some other libraries that are pure-Ruby implementations
- The API is not as intuitive or user-friendly as some other Ruby libraries for parsing XML, which can make it more challenging to use for less experienced developers
- It does not natively support JSON parsing, it will require additional configuration and tools to handle JSON
- It may not handle malformed XML as well as some other libraries.
REXML
REXML is a pure Ruby library for parsing XML documents, it is included with the standard Ruby library, so it's easy to use and doesn't require any additional installation.
Inspired by the Electric XML library for Java, it features an easy-to-use API, a small size, and speed.
Gem Command
gem install rexml
Code Samples
require "rexml/document"
doc = REXML::Document.new(%{
<?xml version="1.0"?>
<Payment>
<Shop>ikea</Shop>
<Amount>199.99</Amount>
<Date>2023-01-12</Date>
</Payment>
})
doc.elements.each("//Shop"){ |element| puts element.text }
Pros & Cons
Pros
- It is included with the standard Ruby library, so it is easy to install and use.
- REXML is pure Ruby, meaning it does not rely on any C libraries or external dependencies, which makes it platform-independent.
- It has a simple and easy-to-use API, which makes it a good choice for small to medium-sized XML documents
- Has a built-in XPath implementation, which makes it easy to search and select elements in an XML document
Cons
- REXML is not as fast as other libraries, like Nokogiri, and it can consume more memory, which makes it not well suited for large XML documents.
- It lacks some of the more advanced features of other XML libraries, like handling XML namespaces, or advanced error handling
Selenium Web Driver
Selenium WebDriver is not primarily a HTML or XML parser, but rather a browser automation tool. It allows you to interact with a web browser programmatically, simulating user actions such as clicking buttons, filling out forms, and navigating between pages.
Selenium WebDriver allows you to automate interactions with web browsers such as clicking buttons, filling out forms, and navigating between pages.
The tool is useful in cases where you need to scrape a website that uses JavaScript to dynamically load its content or to perform specific actions like interacting with a form or a button on the page.
Gem Command
gem install selenium-webdriver webdrivers
Code Samples
require "selenium-webdriver"
require "webdrivers/chromedriver"
driver = Selenium::WebDriver.for :chrome
driver.get("https://webscrapingapi.com")
puts driver.title
Pros & Cons
Here are some of the pros and cons of using Selenium WebDriver in Ruby:
Pros
- Selenium WebDriver supports a wide range of web browsers, including Chrome, Firefox, Edge, Safari and others, which means that the tests that you create can run on different browsers without modification.
- Selenium WebDriver provides a number of ways to inspect the contents of a web page, such as locating elements by their ID, class name, or CSS selector, which makes it easy to interact with web pages and automate tasks.
- It allows you to interact with javascript elements on web pages, this feature makes it suitable for testing the behavior of web pages with javascript.
- It's widely used in the industry and well-documented and has a large community of developers that can provide support.
Cons
- Selenium WebDriver can be slower than other HTML parsing libraries since it needs to launch a browser and simulate a real user's interaction, this can increase the time required to scrape the data.
- Selenium WebDriver depends on a web browser to be installed on the machine, which can cause problems when running the script on a headless environment or on a server without GUI.
- Selenium WebDriver is not a specialized library for HTML parsing and its API might not be as intuitive or user-friendly as specialized libraries like Nokogiri or
Worth Mentioning
Although we have focused on active and well-maintained libraries for parsing HTML and XML in Ruby, there are a few other libraries worth considering.
However, it's important to keep in mind that these libraries may be less actively maintained or have less community support, which can add an additional level of risk if used in a production environment.
It is essential to carefully evaluate the library's features and performance, as well as the size and complexity of the documents you need to parse, before making a decision.
Hpricot
Hpricot is another popular Ruby HTML parser with support for XML documents. Hpricot has a simple and easy-to-use API, and it is well-suited for small to medium-sized documents.
Gem Command
gem install hpricot
Code Samples
require "hpricot"
doc = "<!DOCTYPE html><html><head><title>Hello, World!</title></head><body>Hello, World!</body></html>"
puts Hpricot(doc).at("title").inner_html
Pros & Cons
Here are some pros and cons of using Hpricot:
Pros
- Hpricot has a simple and easy-to-use API that makes it easy to navigate and search through HTML and XML documents.
- Hpricot's search functions are based on jQuery-like CSS selectors, which are easy to understand and use.
- Because some parts of Hpricot are written in C, the library is relatively fast and efficient
- It is suitable for small to medium-sized documents
- Just like Nokogiri, it can parse malformed documents
Cons
- Hpricot has not been actively maintained since 2010, so it might not work well with recent versions of Ruby and it might lack support for new features and bugfixes.
- Hpricot's search functions do not support all CSS selectors, and it does not support XML namespaces.
- It can’t handle malformed XML documents
- Hpricot's performance can be slower and it can consume more memory compared to other libraries like Nokogiri or Ox, particularly for larger documents.
Conclusion
In conclusion, when it comes to parsing HTML and XML documents in Ruby, there are a variety of libraries to choose from, each with their own set of pros and cons.
Nokogiri, REXML, Ox, Hpricot, and LibXML Ruby are all powerful libraries that can be used for web scraping, but it's important to evaluate the specific requirements and needs of your project before deciding which one to use.
Selenium WebDriver, while not primarily designed for HTML parsing, can also be used for web scraping, however it's a browser automation tool that brings some additional complexity and slower performance compared to specialized libraries.
However, building a web scraping script can be a time-consuming and difficult task, especially if you need to handle dynamic websites, CAPTCHAs, and handle bans.
WebScrapingAPI offers a simple and effective solution for obtaining data from the web, eliminating the need to create your own script. Using the Extraction rules feature, you can easily retrieve information from a webpage by specifying the element's CSS selectors.
Why don’t you create an account today?
News and updates
Stay up-to-date with the latest web scraping guides and news by subscribing to our newsletter.
We care about the protection of your data. Read our Privacy Policy.
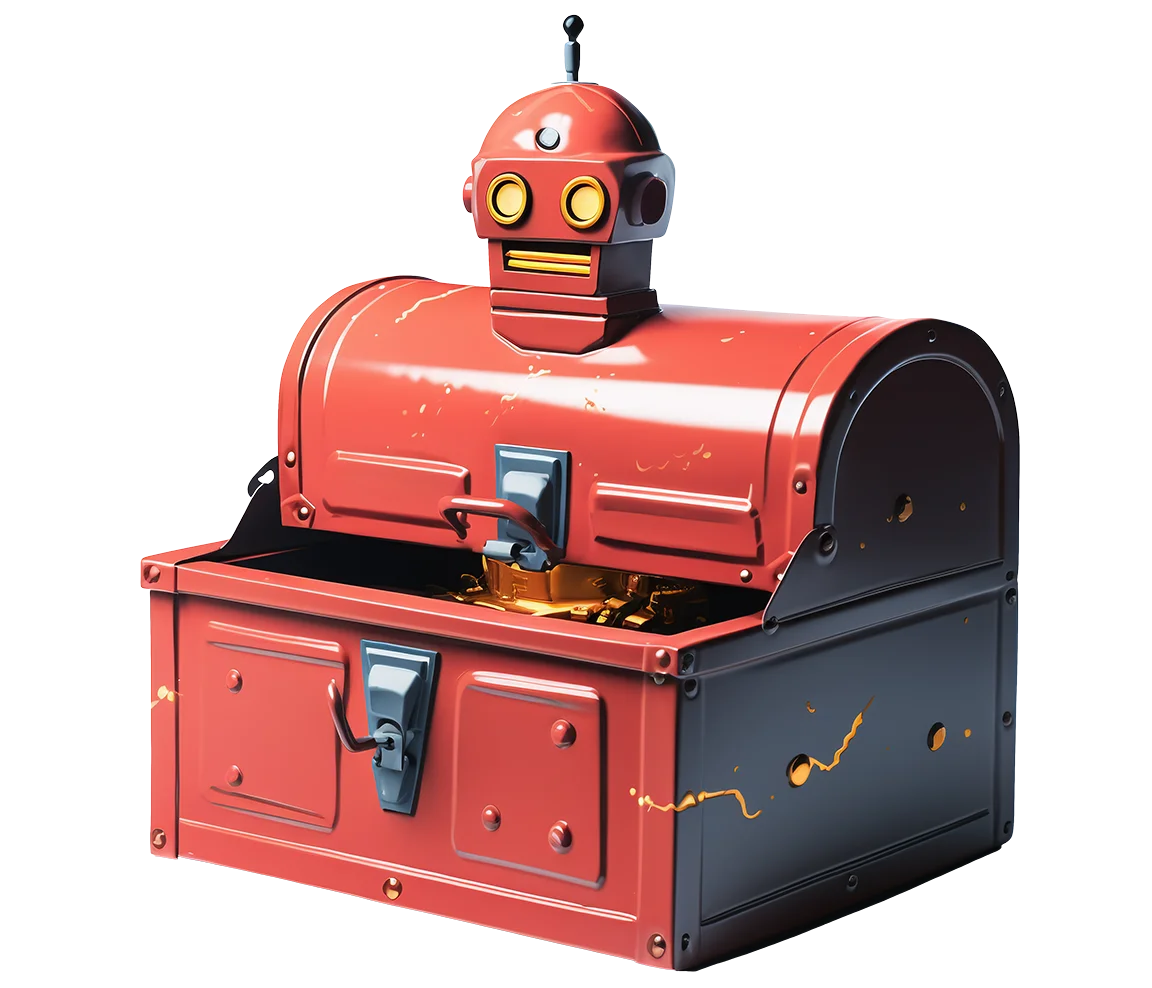
Related articles

Scrape Amazon efficiently with Web Scraping API's cost-effective solution. Access real-time data, from products to seller profiles. Sign up now!


Explore the in-depth comparison between Scrapy and Selenium for web scraping. From large-scale data acquisition to handling dynamic content, discover the pros, cons, and unique features of each. Learn how to choose the best framework based on your project's needs and scale.

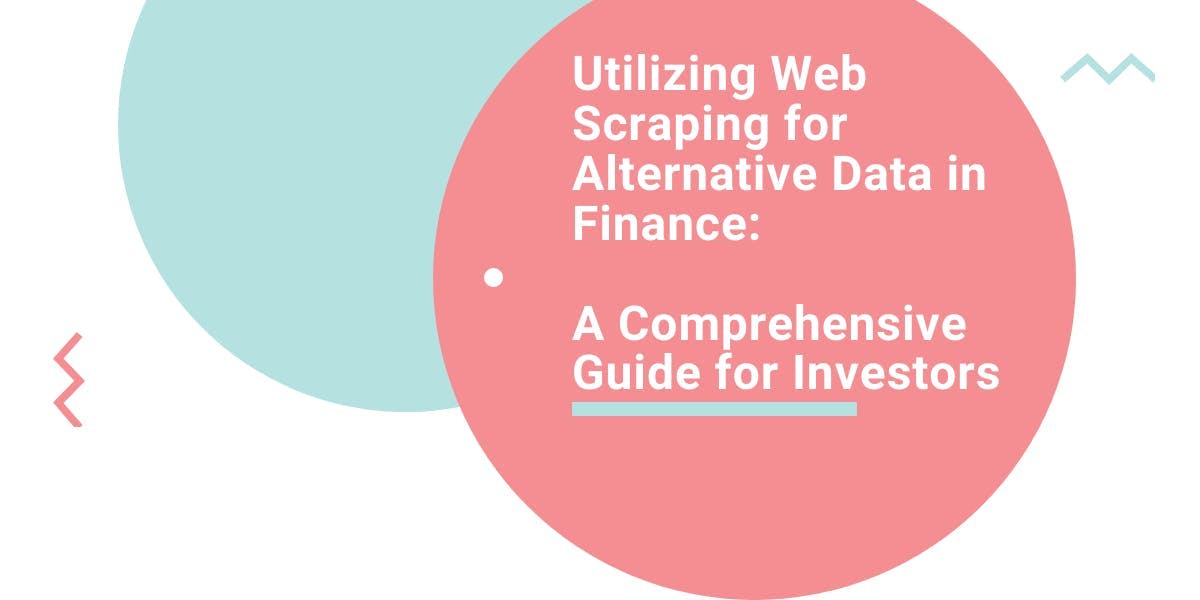
Explore the transformative power of web scraping in the finance sector. From product data to sentiment analysis, this guide offers insights into the various types of web data available for investment decisions.
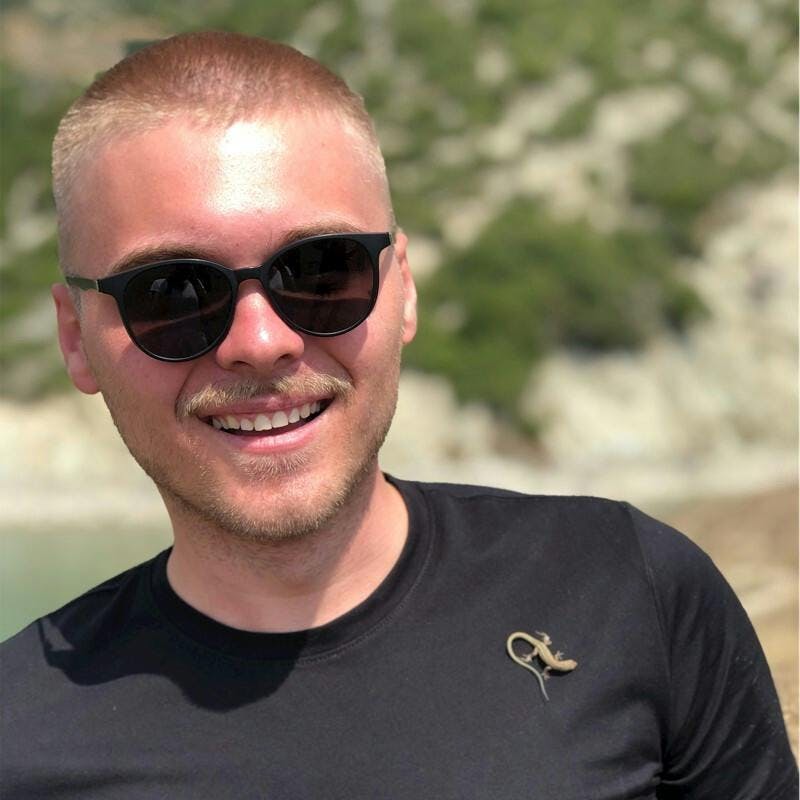