How To Scrape Amazon Product Data: A Comprehensive Guide to Best Practices & Tools
Suciu Dan on Aug 10 2023

Amazon, the global e-commerce giant, is a treasure trove of essential data that includes intricate details like product descriptions, customer reviews, pricing structures, and more. Leveraging this information in a meaningful way is crucial for the contemporary business landscape. Whether your objective is to gauge the performance of products offered by third-party sellers, analyze your competition, or delve into comprehensive market research, utilizing specialized web scraping tools such as Amazon Scraper is essential.
However, the process of scraping Amazon is unique and comes with its own set of challenges and intricacies. This in-depth guide aims to provide a comprehensive overview of each phase required to construct a fully functional Amazon web scraper, allowing you to harness this vital data effectively. It will take you through the specific considerations and techniques tailored for Amazon's complex structure, helping you to navigate the nuances of this powerful platform.
From understanding the legal and ethical aspects of web scraping to providing practical, hands-on steps to create a customized scraping tool, this guide will equip you with the knowledge and tools needed to turn Amazon's vast repository of data into actionable insights for your business.
Preparing for Scraping Amazon
Scraping Amazon is a complex task that requires a set of tools and a strategic approach. Here's a step-by-step guide to prepare your system for scraping Amazon product data.
Step 1: Install Python
Python is the core programming language for web scraping. Ensure that you have Python 3.8 or above installed. If not, head over to python.org to download and install the latest version of Python.
Step 2: Create a Project Folder
Create a dedicated folder to store your code files for web scraping Amazon. Organizing your files will make your workflow smoother.
Step 3: Set Up a Virtual Environment
Creating a virtual environment is considered best practice in Python development. It allows you to manage dependencies specific to the project, ensuring that there's no conflict with other projects.
For macOS and Linux users, execute the following commands to create and activate a virtual environment:
$ python3 -m venv .env
$ source .env/bin/activate
For Windows users, the commands are slightly different:
c:\amazon>python -m venv .env
c:\amazon>.env\scripts\activate
Step 4: Install Required Python Packages
Two primary steps in web scraping are retrieving the HTML and parsing it to extract the relevant data.
- Requests Library: A popular third-party Python library used for making HTTP requests. It offers a simple interface to communicate with web servers but returns HTML as a string, which is not easy to query.
- Beautiful Soup: This Python library helps in web scraping to extract data from HTML and XML files, allowing for searching specific elements like tags, attributes, or text.
Install these libraries using the following command:
$ python3 -m pip install requests beautifulsoup4
Note for Windows users: Replace python3 with python.
Step 5: Basic Scraping Setup
Create a file named amazon.py and insert the code to send a request to a specific Amazon product page. For instance:
import requests
url = 'https://www.amazon.com/Robux-Roblox-Online-Game-Code/dp/B07RZ74VLR/'
response = requests.get(url)
print(response.text)
Running this code might lead to Amazon blocking the request and returning an error 503, as it recognizes that the request was not made through a browser.
Step 6: Overcoming Blocking Mechanisms
Amazon often blocks scraping attempts, returning error codes starting with 400 or 500. To overcome this, you can mimic a browser by sending custom headers, including the user-agent and sometimes accept-language.
Find your browser's user-agent by pressing F12, opening the Network tab, reloading the page, and examining the Request Headers.
Here's an example dictionary for custom headers:
custom_headers = {
'user-agent': 'Mozilla/5.0 (Windows NT 6.1; Win64; x64) AppleWebKit/602.18 (KHTML, like Gecko) Chrome/54.0.3129.163 Safari/602.6 Edge/9.50428',
'accept-language': 'en-US,en;q=0.9',
}
Send this dictionary using the get method like this:
response = requests.get(url, headers=custom_headers)
This will likely yield the desired HTML with product details. Sending as many headers as possible minimizes the need for JavaScript rendering. If rendering is necessary, tools like Playwright or Selenium can be used.
Scraping Amazon Product Data
When it comes to the extraction of product information from Amazon, one typically encounters two primary types of pages that contain the essential data: the category page and the product details page. Each of these plays a crucial role in scraping the information required, and it is vital to know how to navigate both.
The Category Page
Take, for example, the URL https://www.amazon.com/s?i=specialty-aps&bbn=16225007011&rh=n%3A16225007011%2Cn%3A193870011
On the category page, you'll find the basics:
- Product Title: The name of the product.
- Product Image: Visual representation of the item.
- Product Rating: Users' ratings and feedback.
- Product Price: The current selling price.
- Product URLs Page: Links to individual product pages.
Should you require more detailed insights, like the product descriptions or specifications, you'll need to venture into the individual product details pages.
Delving into the Product Details Page
By clicking a product URL (such as https://www.amazon.com/Robux-Roblox-Online-Game-Code/dp/B07RZ74VLR/), you'll enter a treasure trove of detailed information. To see how this information is structured, you can utilize a modern browser like Chrome.
Inspecting HTML Elements
Right-click on the product title, and select "Inspect." You will find the HTML markup of the product title highlighted. In particular, it's contained within a span tag, and its id attribute is defined as "productTitle".
The same method can be used to find the markup of other essential elements:
- Price: Right-click the price, and select "Inspect." The dollar component of the price is housed within a span tag with the class "a-price-whole," while the cents are stored in another span tag, designated with the class "a-price-fraction."
- Rating, Image, and Description: Utilize the same inspect feature to locate these essential components, each wrapped within specific tags and classes.
The process of scraping product data from Amazon can be broken down into specific steps, each targeting a particular aspect of the product information. By employing Python libraries such as requests and BeautifulSoup, we can access, locate, and scrape desired details.
Here's a detailed guide on how to proceed:
1. Initiate the Request
Start by sending a GET request with custom headers to the URL of the product page:
response = requests.get(url, headers=custom_headers)
soup = BeautifulSoup(response.text, 'lxml')
We use BeautifulSoup to parse the HTML content, which facilitates the querying of specific information through CSS selectors.
2. Locate and Scrape Product Name
Identify the product title using the unique id productTitle inside a span element:
title_element = soup.select_one('#productTitle')
title = title_element.text.strip()
3. Locate and Scrape Product Rating
To scrape the product rating, you need to access the title attribute of the #acrPopover selector:
rating_element = soup.select_one('#acrPopover')
rating_text = rating_element.attrs.get('title')
rating = rating_text.replace('out of 5 stars', '')
4. Locate and Scrape Product Price
Extract the product price using the #price_inside_buybox selector:
price_element = soup.select_one('#price_inside_buybox')
print(price_element.text)
5. Locate and Scrape Product Image
Retrieve the default image URL using the #landingImage selector:
image_element = soup.select_one('#landingImage')
image = image_element.attrs.get('src')
6. Locate and Scrape Product Description
Fetch the product description using the #productDescription selector:
description_element = soup.select_one('#productDescription')
print(description_element.text)
7. Locate and Scrape Product Reviews
Scraping reviews is more complex, as one product can have several reviews. A single review may contain various information like author, rating, title, content, date, and verification status.
Collecting Reviews
Use the div.review selector to identify and collect all the reviews:
review_elements = soup.select("div.review")
scraped_reviews = []
for review in review_elements:
# Extracting specific review details...
Extracting Review Details
Each review can be dissected into specific details:
- Author: span.a-profile-name
- Rating: i.review-rating
- Title: a.review-title > span:not([class])
- Content: span.review-text
- Date: span.review-date
- Verified Status: span.a-size-mini
Each of these elements can be selected using their respective CSS selectors and then extracted using methods similar to the previous steps.
Assembling the Review Data
Create an object containing the extracted review details and append it to the array of reviews:
r = {
"author": r_author,
"rating": r_rating,
"title": r_title,
"content": r_content,
"date": r_date,
"verified": r_verified
}
scraped_reviews.append(r)
Scraping Amazon product data is a multifaceted task that requires a precise approach to target specific elements within the web page's structure. By leveraging the capabilities of modern web scraping tools, it is possible to successfully extract detailed product information.
Handling Product Listing
To scrape detailed product information, you'll often start from a product listing or category page, where products are displayed in a grid or list view.
Identifying Product Links
On a category page, you might notice that each product is contained within a div with a specific attribute [data-asin]. The links to individual products are often found inside an h2 tag within this div.
The corresponding CSS selector for these links would be:
[data-asin] h2 a
Parsing and Following Links
You can use BeautifulSoup to select these links and extract the href attributes. Note that these links may be relative, so you'll want to use the urljoin method to convert them to absolute URLs.
from urllib.parse import urljoin
def parse_listing(listing_url):
# Your code to fetch and parse the page goes here...
link_elements = soup_search.select("[data-asin] h2 a")
page_data = []
for link in link_elements:
full_url = urljoin(listing_url, link.attrs.get("href"))
product_info = get_product_info(full_url)
page_data.append(product_info)
Handling Pagination
Many listing pages are paginated. You can navigate to the next page by locating the link that contains the text "Next."
next_page_el = soup.select_one('a:contains("Next")')
if next_page_el:
next_page_url = next_page_el.attrs.get('href')
next_page_url = urljoin(listing_url, next_page_url)
You can then use this URL to parse the next page, continuing the loop until there are no more "Next" links.
8. Export Scraped Product Data to a JSON File
The scraped product data is being collected as dictionaries within a list. This format allows for easy conversion to a Pandas DataFrame, facilitating data manipulation and export.
Here's how you can create a DataFrame from the scraped data and save it as a JSON file:
import pandas as pd
df = pd.DataFrame(page_data)
df.to_json('baby.json', orient='records')
This will create a JSON file containing all the scraped product information.
This guide provides a step-by-step walkthrough of scraping product listings, including navigation through pagination and exporting the results to a JSON file. It's essential to tailor these methods to the specific structure and requirements of the site you're scraping.
Full Code
import requests
from bs4 import BeautifulSoup
from urllib.parse import urljoin
import pandas as pd
custom_headers = {
"accept-language": "en-US,en;q=0.9",
"user-agent": "{user-agent}",
}
def get_response(url):
"""Make a GET request and return the response if successful."""
with requests.Session() as session:
session.headers.update(custom_headers)
response = session.get(url)
if response.status_code != 200:
print(f"Error in getting webpage {url}")
return None
return response
def get_product_info(url):
"""Scrape product details from the given URL."""
response = get_response(url)
if response is None:
return None
# ... rest of the code ...
return {
"title": title,
"price": price,
"rating": rating,
"image": image,
"description": description,
"url": url,
"reviews": scraped_reviews,
}
def parse_listing(listing_url):
"""Parse multiple product listings from the given URL."""
page_data = []
while listing_url:
response = get_response(listing_url)
if response is None:
break
soup_search = BeautifulSoup(response.text, "lxml")
link_elements = soup_search.select("[data-asin] h2 a")
for link in link_elements:
full_url = urljoin(listing_url, link.attrs.get("href"))
print(f"Scraping product from {full_url[:100]}", flush=True)
product_info = get_product_info(full_url)
if product_info:
page_data.append(product_info)
next_page_el = soup_search.select_one('a:contains("Next")')
listing_url = urljoin(listing_url, next_page_el.attrs.get('href')) if next_page_el else None
print(f'Scraping next page: {listing_url}', flush=True) if listing_url else None
return page_data
def main():
search_url = "{category url}"
data = parse_listing(search_url)
df = pd.DataFrame(data)
df.to_json("amz.json", orient='records')
if __name__ == '__main__':
main()
Best Practices and Techniques
Scraping data from Amazon is not as straightforward as it might seem. With the increasing complexity of web security, extracting valuable information from this colossal e-commerce platform presents a myriad of challenges. From rate-limiting to intricate bot-detection algorithms, Amazon ensures it remains a challenging target for data scraping.
Challenges in Amazon Data Scraping
- Rate-Limiting: Amazon enforces rate-limiting measures to control the number of requests from a single IP address. Exceeding these limits can result in your IP being blocked.
- Bot-Detection Algorithms: Sophisticated algorithms are in place to inspect your HTTP headers for unusual patterns, checking if the requests come from automated bots.
- Constantly Changing Layouts: With various page layouts and fluctuating HTML structures, keeping up with the ever-changing interface requires vigilance and adaptability.
Strategies to Overcome the Challenges
Navigating these obstacles necessitates a strategic approach. Here are some essential best practices to follow when scraping Amazon:
- Utilize a Realistic User-Agent: Making your User-Agent appear genuine is crucial in bypassing detection. Here are the most prevalent user agents that mimic real browser activity.
- Set Your Fingerprint Consistently: Many platforms, including Amazon, use Transmission Control Protocol (TCP) and IP fingerprinting to identify bots. Ensuring that your fingerprint parameters remain uniform is vital in staying under the radar.
- Alter the Crawling Pattern Thoughtfully: Crafting a successful crawling pattern involves simulating how a genuine user would navigate through a webpage. This includes incorporating clicks, scrolls, and mouse movements that mimic human behavior. Designing a pattern that mirrors human interaction can reduce the likelihood of detection.
- Consider Proxy Management: While the initial article doesn’t mention this, using proxies can add an extra layer of anonymity. By distributing requests across various IP addresses, you can further evade detection.
- Stay Updated with Amazon’s Policies and Technologies: Amazon frequently updates its security measures and user interface. Regularly revisiting and adapting your scraping methods to these changes will ensure your techniques remain effective.
The task of scraping Amazon product data is intricate, demanding an in-depth understanding of the best practices and constant adaptation to Amazon's evolving strategies. By embracing these techniques and staying vigilant to the ever-changing landscape, you can access the valuable data needed for your analysis or project. Remember, this is just a surface glance at what's required when scraping Amazon, and additional research and tools may be necessary to achieve your specific objectives.
An Effortless Way to Extract Amazon Data: Utilizing Amazon Scraper API
While the manual scraping methods detailed above can certainly yield valuable insights, they require continuous monitoring, adaptation, and technical know-how. For those seeking a more streamlined and user-friendly approach, the Amazon Scraper API offers an efficient and dedicated solution.
Why Choose Amazon Scraper API?
Amazon Scraper API is a purpose-built tool specifically designed for navigating the complexities of scraping Amazon. Here's what you can achieve with this specialized API:
- Versatile Scraping Options: You can scrape and parse various Amazon page types. Whether you are looking to extract data from Search, Product, Offer Listing, Questions & Answers, Reviews, Best Sellers, or Sellers pages, this API has you covered.
- Global Reach: Target and retrieve localized product data across an impressive 195 locations worldwide. This vast coverage allows for robust analysis and insights into different markets and demographics.
- Efficient Data Retrieval: The API returns accurate parsed results in a clean JSON format. There's no need for additional libraries or complex configurations; you receive the data ready for immediate use.
- Enhanced Features for Advanced Needs: Enjoy features tailored for efficiency, such as bulk scraping capabilities and automated jobs. These functionalities streamline the scraping process, enabling you to gather vast amounts of data with minimal manual intervention.
- Compliance and Ease of Use: Unlike manual scraping, using a dedicated API like Amazon Scraper API often ensures better compliance with legal regulations and Amazon's terms of service, making it a more secure option for data extraction.
Conclusion
Extracting Amazon product data can be approached through two distinct methods, each catering to different skill sets and requirements. Let's explore both avenues:
Crafting Your Own Scraper with Requests and Beautiful Soup
If you're inclined towards coding and possess the necessary skills, creating a custom scraper using popular Python libraries like Requests and Beautiful Soup can be an intriguing venture. Here's a brief overview of the process:
Sending Custom Headers: By customizing HTTP headers, you can mimic genuine browser requests and evade detection.
Rotating User-Agents: Frequent changes to the User-Agent can further disguise your scraping activities, making them appear more like ordinary user interactions.
Proxy Rotation: Utilizing a pool of proxies enables you to distribute requests across multiple IP addresses, helping to bypass bans or rate limiting.
While this method offers flexibility and control, it demands significant effort, time, and continuous monitoring. Amazon's ever-changing layout and strict anti-bot measures make this a challenging path, requiring constant updates and fine-tuning.
Streamlined Solution with Amazon Scraper API
For those seeking a more user-friendly and time-efficient alternative, Amazon Scraper API provides a tailor-made solution:
- Pre-Built Functionality: The API is specifically designed for Amazon, offering features to scrape various page types with ease.
- Comprehensive Coverage: With the ability to target data in numerous global locations, the API is versatile and far-reaching.
- Ease of Use: Forget the complexities of manual coding; the API returns ready-to-use data in a convenient JSON format.
Amazon Scraper API represents an accessible entry point to Amazon data scraping, especially for individuals or organizations lacking the technical resources or the time to develop and maintain a custom scraper.
Whether you choose to write your own code with Requests and Beautiful Soup or opt for the specialized Amazon Scraper API, your decision should align with your skills, resources, goals, and compliance with legal and ethical guidelines.
- For tech-savvy users who relish a challenge, coding a custom scraper offers control and customization.
- For those prioritizing efficiency, accessibility, and compliance, Amazon Scraper API provides a ready-made solution that simplifies the process.
Both paths can lead to valuable insights, but your choice will significantly impact the journey. Understanding the strengths and limitations of each approach will help you make an informed decision that best suits your needs.
FAQ
Does Amazon Allow Scraping?
Scraping publicly available information from Amazon is generally not deemed illegal, but it must be in compliance with Amazon's Terms of Service (ToS). However, this is a complex legal area. Before proceeding, consult with legal professionals who specialize in this field to ensure that your specific scraping activities are lawful.
Can Scraping Be Detected?
Yes, scraping can indeed be detected. Many websites, including Amazon, use anti-bot software that examines various factors, such as your IP address, browser parameters, and user agents. If suspicious activity is detected, the site may present a CAPTCHA challenge, and continued detection could lead to your IP being blocked.
Does Amazon Ban IP Addresses?
Yes, Amazon may ban or temporarily block an IP address if it identifies it as suspicious or in violation of its anti-bot measures. It's an essential part of their security protocols to protect the integrity of the platform.
How Can I Bypass CAPTCHA While Scraping Amazon?
Bypassing CAPTCHAs is one of the significant obstacles in data scraping, and avoiding them altogether is preferable. Here's how you might minimize encounters:
- Utilize reliable proxies and consistently rotate your IP addresses.
- Introduce random delays between requests to mimic human behavior.
- Ensure that your fingerprint parameters are consistent.
It's worth noting that CAPTCHA handling might require ethical consideration, and following best practices is advised.
How Can I Crawl Amazon?
Amazon's complex structure can be navigated using specialized scraping tools. While you can utilize free web scraping and crawling tools like Scrapy, these may require substantial effort to set up and maintain.
For a more effortless and efficient solution, you might consider using a dedicated service like Amazon Scraper API. Such tools are designed specifically to deal with the intricacies of Amazon and can greatly simplify the crawling process.
News and updates
Stay up-to-date with the latest web scraping guides and news by subscribing to our newsletter.
We care about the protection of your data. Read our Privacy Policy.
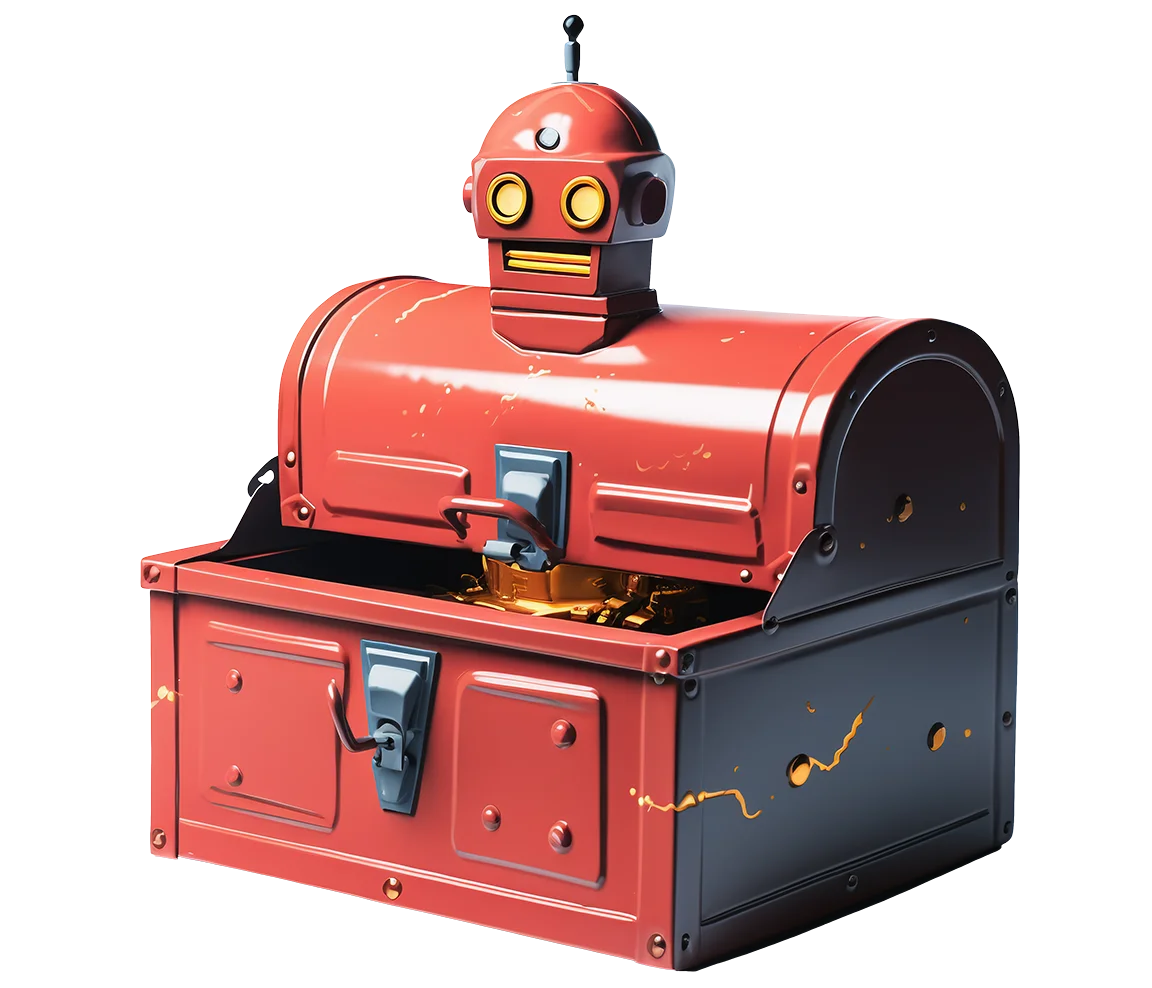
Related articles

Scrape Amazon efficiently with Web Scraping API's cost-effective solution. Access real-time data, from products to seller profiles. Sign up now!


Explore the in-depth comparison between Scrapy and Selenium for web scraping. From large-scale data acquisition to handling dynamic content, discover the pros, cons, and unique features of each. Learn how to choose the best framework based on your project's needs and scale.

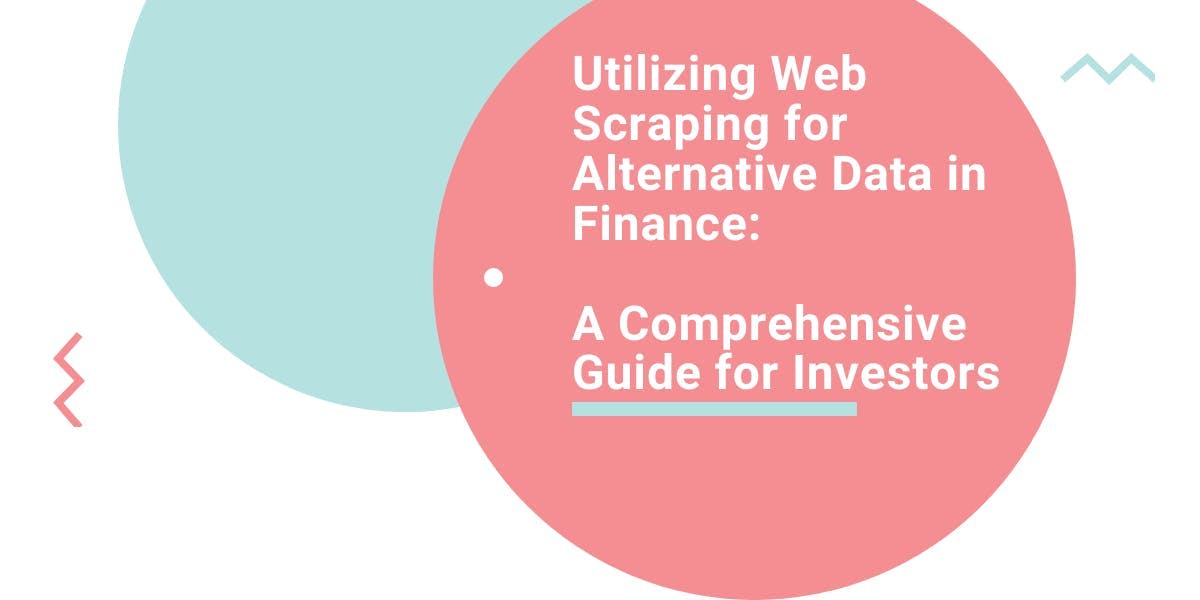
Explore the transformative power of web scraping in the finance sector. From product data to sentiment analysis, this guide offers insights into the various types of web data available for investment decisions.
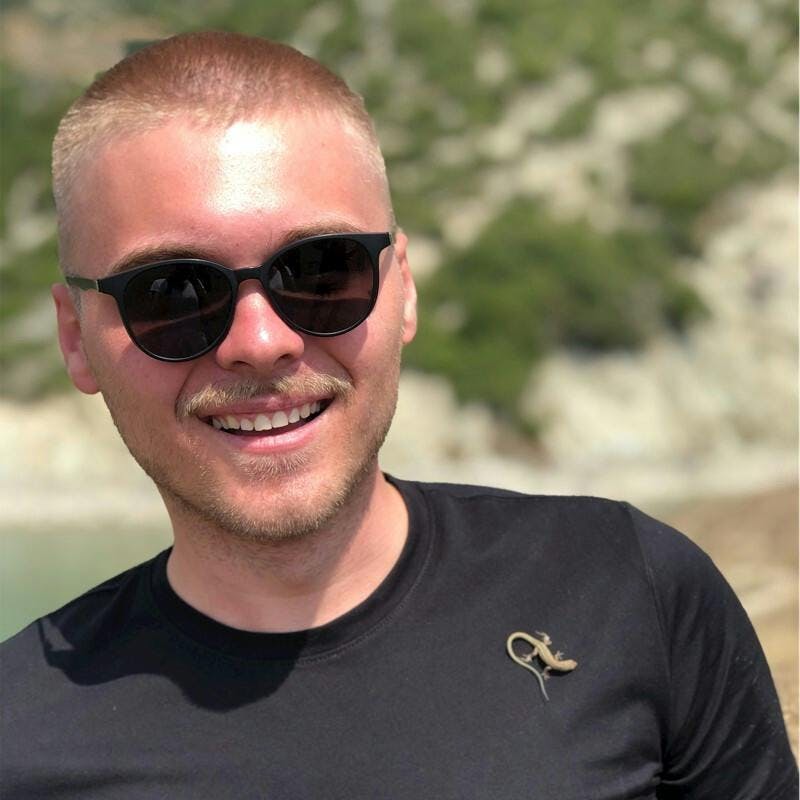